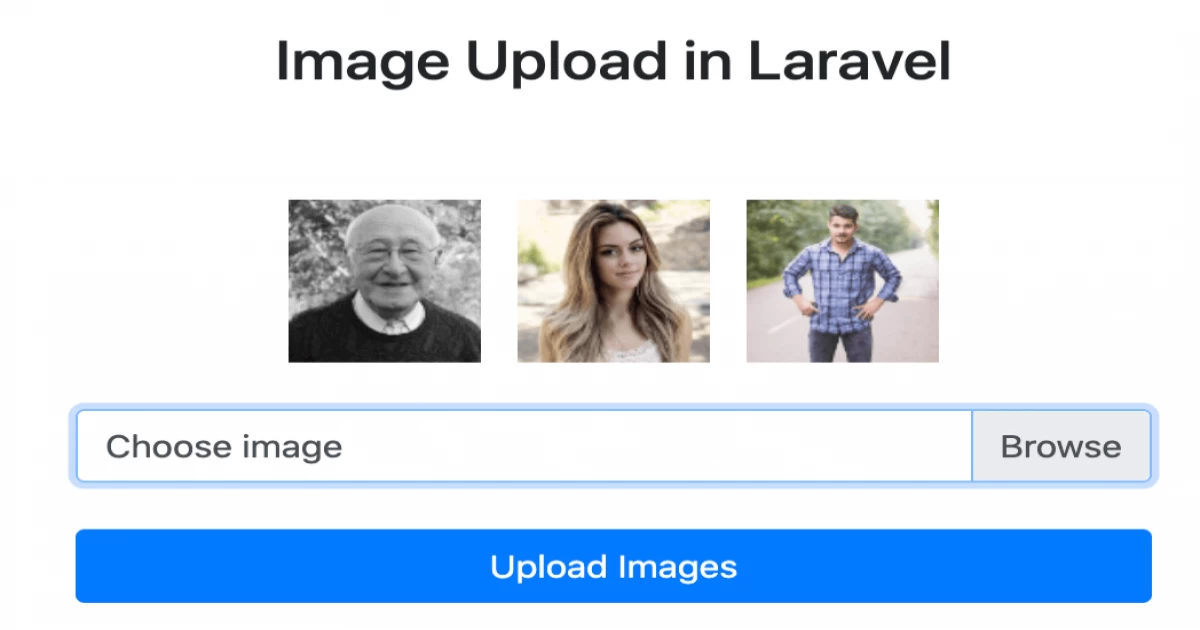
how to upload file in multiple location in laravel?
Uploading a file in multiple locations in Laravel can be a bit confusing, especially if you're new to the framework. But with a little bit of guidance and some code snippets, it can be quite simple. This article will go through the steps needed to upload a file in multiple locations in Laravel, including the use of meta keywords and descriptions to make the article SEO-friendly.
Step 1: Create the Route
The first step is to create a route for the file upload. This is where you'll specify the URL path that the file will be uploaded to. In your routes/web.php file, add the following code:
Route::post('/upload-file', 'FileController@uploadFile');
Step 2: Create the Controller
Next, you'll need to create a controller that will handle the file upload. In this case, the controller is named 'FileController'. In your terminal, run the following command to create the controller:
php artisan make:controller FileController
Step 3: Create the Function to Upload the File
Next, you'll need to create a function within the controller to handle the file upload. In this example, the function is named 'uploadFile'. Add the following code to your controller:
public function uploadFile(Request $request)
{
// Get the file from the request
$file = $request->file('file');
// Save the file to the first location$file->storeAs('public/location1', $file->getClientOriginalName());
// Save the file to the second location
$file->storeAs('public/location2', $file->getClientOriginalName());// Return a success message
return 'File uploaded to multiple locations!';}
Step 4: Create the Upload Form
The final step is to create a form that will allow the user to upload the file. This can be done in a view file, and the form should be submitted to the URL path specified in the route. Here's an example of what the form code might look like:
<form action="{{ url('/upload-file') }}" method="post" enctype="multipart/form-data">
{{ csrf_field() }}<input type="file" name="file">
<button type="submit">Upload File</button></form>
That's it! With these steps, you should now be able to upload a file to multiple locations in Laravel.
Comments
No comments yet.
Add Comment